Best Advice for New Developers: Focus on Frameworks, Not Just Core Languages
As a new developer, you might be tempted to learn every little detail of programming languages like HTML, CSS, PHP, or JavaScript before diving into frameworks. But here’s the truth: frameworks are your best friend when it comes to building real-world applications quickly and efficiently.
I spent my first three months learning the fundamentals, but quickly realized that frameworks were designed to fix the issues and limitations of base languages. They make development faster, cleaner, and more maintainable — something i
Best Advice for New Developers: Focus on Frameworks, Not Just Core Languages
As a new developer, you might be tempted to learn every little detail of programming languages like HTML, CSS, PHP, or JavaScript before diving into frameworks. But here’s the truth: frameworks are your best friend when it comes to building real-world applications quickly and efficiently.
I spent my first three months learning the fundamentals, but quickly realized that frameworks were designed to fix the issues and limitations of base languages. They make development faster, cleaner, and more maintainable — something indie developers or solo projects need. Here’s why:
1. HTML/CSS: Don't Write Everything from Scratch
Writing raw HTML and CSS can be time-consuming, error-prone, and hard to maintain. Thankfully, frameworks like Bootstrap and Tailwind CSS simplify the process.
- Bootstrap offers pre-designed components and grids that help you build responsive, professional websites with minimal effort.
- Tailwind CSS uses a utility-first approach, allowing you to apply classes directly in your HTML, leading to faster customization and less CSS to write.
These frameworks not only save time but also make your code more organized and maintainable. You don’t need to reinvent the wheel for every project. Focus on building features rather than spending hours coding styles.
2. PHP: Let Laravel Handle the Heavy Lifting
I struggled early on with inserting multiple data entries in PHP and interacting with MySQL. But once I switched to Laravel, everything changed. Laravel is a PHP framework that simplifies tasks like routing, database interaction, and authentication.
- Eloquent ORM: Makes working with databases easier and cleaner by allowing you to interact with your data using simple PHP syntax.
- Blade Templating: Streamlines views and layouts, making it easier to build dynamic websites.
Laravel provides all the tools you need to avoid the repetitive tasks and complex boilerplate code that come with plain PHP. It’s perfect for indie developers who want to focus on logic rather than constantly troubleshooting.
3. JavaScript: Combining jQuery and React for Flexibility
JavaScript is a powerful language, but managing DOM manipulation and state can be tedious without the right tools. I recommend using jQuery for simple, DOM-based tasks, and React for building complex, dynamic user interfaces.
- jQuery is lightweight, easy to learn, and works great for small projects or handling basic animations.
- React is ideal for larger applications that require state management and reusable components. It's the go-to framework for modern web development.
By combining these tools, you can quickly scale from simple features to more sophisticated applications without getting bogged down in complex JavaScript code.
Key Takeaways for New Developers:
- Don't reinvent the wheel: Use frameworks to save time and effort. They address common issues and help you write cleaner, more maintainable code.
- Focus on building projects: Instead of getting stuck on the basics of each language, use frameworks to focus on delivering functional apps faster.
- Choose the right tools: Find frameworks that match your needs and the scale of your project. Don’t hesitate to switch to a framework that better suits your development style.
My Top Framework Recommendations:
- HTML/CSS: Bootstrap, Tailwind CSS
- PHP: Laravel
- JavaScript: jQuery (for small tasks), React (for larger, interactive apps)
Frameworks aren’t shortcuts, they’re the right tools for getting things done quickly and efficiently. By mastering frameworks, you can build better applications in less time, which will make you more productive and more confident in your development journey.
So, focus on frameworks, and start building real-world applications today!
When it comes to web development, even the smallest tweaks can make a big difference. Whether you’re optimizing user experiences or creating delightful interactions, there are clever tricks in HTML, JavaScript, and CSS that often fly under the radar. Here are some interesting hacks you might not know about, explained in a way that’s easy to grasp.
1. CSS :has()
Selector: Style Parent Elements Based on Children
Imagine trying to style a parent element based on the presence of a child element. For years, this was impossible in pure CSS—until now.
- Example: Highlight a card if it contains an image.
- .c
When it comes to web development, even the smallest tweaks can make a big difference. Whether you’re optimizing user experiences or creating delightful interactions, there are clever tricks in HTML, JavaScript, and CSS that often fly under the radar. Here are some interesting hacks you might not know about, explained in a way that’s easy to grasp.
1. CSS :has()
Selector: Style Parent Elements Based on Children
Imagine trying to style a parent element based on the presence of a child element. For years, this was impossible in pure CSS—until now.
- Example: Highlight a card if it contains an image.
- .card:has(img) {
- border: 2px solid blue;
- }
- Why It’s Cool: This simplifies dynamic styling that previously required JavaScript.
2. Smooth Scrolling Without JavaScript
Want to add smooth scrolling to anchor links? You don’t need a single line of JavaScript.
- Example:
- html {
- scroll-behavior: smooth;
- }
- Why It’s Useful: It’s easy to implement and enhances user experience.
3. Use JavaScript's IntersectionObserver
for Lazy Loading
Instead of relying on libraries, you can use the native IntersectionObserver
API to load images or content only when they enter the viewport.
- Example:
- const images = document.querySelectorAll('img');
- const observer = new IntersectionObserver((entries) => {
- entries.forEach(entry => {
- if (entry.isIntersecting) {
- entry.target.src = entry.target.dataset.src;
- observer.unobserve(entry.target);
- }
- });
- });
- images.forEach(img => observer.observe(img));
- Why It’s Cool: Saves bandwidth and improves page performance.
4. CSS clip-path
for Creative Shapes
Add visual interest to your designs by cropping elements into custom shapes.
- Example: Create a hexagonal image.
- .image {
- clip-path: polygon(50% 0%, 100% 25%, 100% 75%, 50% 100%, 0% 75%, 0% 25%);
- }
- Why It’s Creative: Adds flair to your designs without needing external assets.
5. Custom Properties (var()
) for Dynamic Theming
CSS variables can make real-time theming ridiculously easy.
- Example: Switch between light and dark modes.
- :root {
- --bg-color: white;
- --text-color: black;
- }
- [data-theme="dark"] {
- --bg-color: black;
- --text-color: white;
- }
- body {
- background-color: var(--bg-color);
- color: var(--text-color);
- }
- Why It’s Powerful: Centralizes theming logic and reduces code duplication.
6. DOM ContentEditable for Quick Prototypes
The contenteditable
attribute allows you to turn any element into an editable field.
- Example:
- <div contenteditable="true">Edit me!</div>
- Why It’s Handy: Great for prototyping or quick inline editing features.
7. Use HTML <dialog>
for Native Modals
Instead of building modals from scratch, use the <dialog>
element for native support.
- Example:
- <dialog id="myDialog">This is a modal!</dialog>
- <button onclick="document.getElementById('myDialog').showModal()">Open Modal</button>
- Why It’s Easy: Handles accessibility and functionality out of the box.
Conclusion: Little Tricks, Big Impact
These hacks may not revolutionize your development workflow, but they can save time, improve user experience, and make your projects more efficient. The key is knowing when to use them—balance is essential. Overusing hacks can lead to messy or less maintainable code.
Pro Tip: Experiment with these hacks in small projects to understand their behavior before applying them to production.
What’s your favorite little-known web dev trick? Share it below!
When you are starting a website, it is not only important to think about all the flow and the process of its creation but also the price it will cost you.
Depending on the website, you can plan the budget beforehand so no unexpected expenses would stand in your way. That will save you time and money.
Starting a website can cost from nothing to as much as you can invest in it. And both cases might not be the best thing that you can do for your own website’s good.
A no-cost website
Despite the fact that time is money, it is possible to build your website for free.
If you are just starting a website a
When you are starting a website, it is not only important to think about all the flow and the process of its creation but also the price it will cost you.
Depending on the website, you can plan the budget beforehand so no unexpected expenses would stand in your way. That will save you time and money.
Starting a website can cost from nothing to as much as you can invest in it. And both cases might not be the best thing that you can do for your own website’s good.
A no-cost website
Despite the fact that time is money, it is possible to build your website for free.
If you are just starting a website and you don’t know if you want to develop it yourself, a free of cost website sounds like a fabulous idea.
There are some hosts that offer hosting services for free, and their purpose is to serve as a learning and testing material.
By trying a free hosting out, you will know if you can create a website on your own or need to find someone who can take care of the technical parts.
Just keep in mind that free hosting isn’t for growing your website on it - it has limited features and, unfortunately, limited resources.
A beta version of your site can be tested there; however, it might be that not all of the functions will be working here too.
I recommend 000webhost.com as a free hosting platform for those who want to try out hosting before buying it and learn some new things.
Although, if you already know what hosting looks like and you want to start your website immediately, don’t waste your time with the free one and grab the best deal you can find in the market!
A low-cost website - from $90 up to 200$ in 4 years
Starting your website probably won’t require many resources, and it’s better to start it slow.
If you are not planning to get a lot of traffic at the beginning and a lot of resources aren’t your priority, you should be looking for shared hosting.
Besides, shared hosting doesn’t require a lot of technical knowledge, so you will be able to save some money by building a website on your own.
From what Hostinger suggests for a start, shared hosting prices can range from $1.99/mo to $3.99/mo (while purchasing the 48 months plan).
So, the price depends on how much you are going to invest in your website and for how long you are going to stick to it.
With Hostinger the highest plan can be billed for a maximum of $191.52 for four years. Not that bad, right?
Besides the hosting plan, there are other parts of the website that will require some expenses too.
Let’s start with the essential one - domain name. It is usually up to $10 per year and worth investing in!
A catchy domain name makes your website visible among the others, and a paid domain name gives it some authority that will rank better in the search engines.
Even if you are planning to spend as little money as possible, don’t forget that the expenses might not stop with the hosting and the domain.
It is truly worth to invest in website security, appearance and getting visitors, but you can also start a website without any additional costs.
In this case, be aware that not investing money in it might make you spend a lot of time on it.
A higher investment website - from $470 up to $2000 in 4 years
From here, the prices start to vary according to the website size, purpose and additional features you are using.
Usually, the websites that need a more significant investment are e-commerce stores, lead generation sites, corporate websites and high-traffic projects.
And for those, it all adds up: the hosting, the domain, the maintenance, the theme of the website, its marketing strategy, or even CMS.
For the higher investment websites, Hostinger suggests cloud hosting plans. There the price starts from $9.99/mo (while purchasing the 48 months plan).
With cloud hosting, a website gets a dedicated IP and it gives the website better stability as its uptime doesn’t depend on the other websites.
In addition to that, these plans are built-up for e-commerce and have many more resources, so in this case, a cloud plan might be better for you than any shared.
Besides, if you are good at developing or you are going to hire a developer, you might want to get a VPS and host your website on a virtual and super private server.
Is it worth to invest in it? For sure!
VPS can give you the freedom and more possibilities to start a website of your dreams.
Small tip: if you are willing to invest in your website, I would recommend investing in hosting first.
User experience highly depends on how fast your website is working and the right hosting plan has a lot to do with the speed and uptime.
Not sure if you know what your website needs? Don’t be afraid to ask for advice!
A lot of companies have their customer support open 24/7 and I’m sure that they might be able to suggest the most suitable option for you.
This might (not) cost you
As I mentioned before, when planning the budget of your website there are more things to consider than the hosting and the domain.
Here the price only depends on your needs and what alternatives you are able to find:
- Marketing strategy. The website’s traffic usually doesn’t grow without any costs or effort. It doesn’t mean that you have to pay for the traffic, surely you can save money by using low-cost strategies that sometimes turn out to be even more effective than expensive ones. However, you should understand that marketing will cost you and the easiest way to make it less painful - planning a long-term strategy.
- Control panel, the site builder, themes and plugins. If you are staying with shared or cloud hosting, you don’t have to worry about the control panel or the builder as they are already implemented in your plan. However, if you have decided to get VPS - keep in mind that some of the control panels can cost you money. Not only that, even if you are planning to use WordPress for building your website, don’t forget that some exclusive themes or plugins might cost you too.
- Developers or other people that could be working for your website and business. Didn’t thought of them while planning the budget? Human resources are the most precious and expensive ones. If your website is an online store and you don’t have any development skills, it might take a while until you are ready to welcome your customers to the site. The best way of dealing with serious business - hiring serious professionals.
- Additional features. These features can start with the Extended Validation SSL certificate, Premium CloudFlare and end up with external email services. Any additional features that might be useful to keep your business at the highest level might cost you more than you could expect at first. If you want to play it safe, having some savings for unplanned expenses is always recommended as well as having a second thought about the features.
That’s all from me! To conclude, starting a website is not so pricey if you know where you can save money, so it is better to start saving with planning all along.
If you have any questions or more ideas regarding budget planning, feel free to leave a comment below.
Have a fun start!
- * { background-color: rgba(255,0,0,.2); }
- * * { background-color: rgba(0,255,0,.2); }
- * * * { background-color: rgba(0,0,255,.2); }
- * * * * { background-color: rgba(255,0,255,.2); }
- * * * * * { background-color: rgba(0,255,255,.2); }
- * * * * * * { background-color: rgba(255,255,0,.2); }
This is one of my favourite inventions.
But what does this horrible thing do?
It is meant to be used when you are working with layout, e.g. take http://time.com.
The problem is that unless the element on the page has a solid background or it is a picture, you do not see how does it fit into the layout, e.g. most of the
- * { background-color: rgba(255,0,0,.2); }
- * * { background-color: rgba(0,255,0,.2); }
- * * * { background-color: rgba(0,0,255,.2); }
- * * * * { background-color: rgba(255,0,255,.2); }
- * * * * * { background-color: rgba(0,255,255,.2); }
- * * * * * * { background-color: rgba(255,255,0,.2); }
This is one of my favourite inventions.
But what does this horrible thing do?
It is meant to be used when you are working with layout, e.g. take http://time.com.
The problem is that unless the element on the page has a solid background or it is a picture, you do not see how does it fit into the layout, e.g. most of the text nodes, pictures with transparency, etc.
With the above CSS, you will see something along the lines of:
Different depth of nodes will use different colour allowing you to see the size of each element on the page, their margin and their padding. Now you can easily identify inconsistencies.
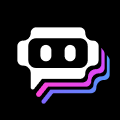
Here are some interesting HTML, JavaScript, DOM, and CSS hacks that many web developers may not be aware of:
- Scroll-linked Animations: Using the
IntersectionObserver
API, you can create scroll-linked animations that are triggered as the user scrolls the page. This allows for more dynamic and engaging experiences.
- const observer = new IntersectionObserver((entries) => {
- entries.forEach((entry) => {
- if (entry.isIntersecting) {
- entry.target.classList.add('animate');
- } else {
- entry.target.classList.remove('animate');
- }
- });
- });
- const elements = document.querySelectorAll('.scroll-li
Here are some interesting HTML, JavaScript, DOM, and CSS hacks that many web developers may not be aware of:
- Scroll-linked Animations: Using the
IntersectionObserver
API, you can create scroll-linked animations that are triggered as the user scrolls the page. This allows for more dynamic and engaging experiences.
- const observer = new IntersectionObserver((entries) => {
- entries.forEach((entry) => {
- if (entry.isIntersecting) {
- entry.target.classList.add('animate');
- } else {
- entry.target.classList.remove('animate');
- }
- });
- });
- const elements = document.querySelectorAll('.scroll-linked');
- elements.forEach((el) => observer.observe(el));
- Aspect Ratio Locking: With the
aspect-ratio
CSS property, you can lock the aspect ratio of an element, which is useful for responsive design and maintaining image proportions.
- .locked-aspect {
- aspect-ratio: 16 / 9;
- }
- Smooth Scrolling with CSS: Instead of using JavaScript to create smooth scrolling, you can achieve the same effect with the
scroll-behavior
CSS property.
- html {
- scroll-behavior: smooth;
- }
- CSS Counters: CSS counters allow you to create dynamic numbering systems, such as ordered lists or step-by-step tutorials, without the need for additional markup.
- body {
- counter-reset: section;
- }
- h2::before {
- counter-increment: section;
- content: "Step " counter(section) ": ";
- }
- Unique Selectors with the : The CSS
:has()
pseudo-class allows you to select elements based on the presence of other elements within them, enabling more complex and specific targeting.
- /* Select a paragraph that has an image as a child */
- p:has(img) {
- background-color: #f0f0f0;
- }
- Conditional CSS with the : The
:is()
pseudo-class can be used to apply styles based on the element type, making your CSS more readable and maintainable.
- :is(h1, h2, h3) {
- font-family: 'Arial', sans-serif;
- }
- Nested Variables with CSS Modules: With CSS Modules, you can create nested variables to better organize and manage your styles.
- .card {
- --card-bg: #ffffff;
- --card-text: #333333;
- background-color: var(--card-bg);
- color: var(--card-text);
- .header {
- --card-header-bg: #f0f0f0;
- --card-header-text: #666666;
- background-color: var(--card-header-bg);
- color: var(--card-header-text);
- }
- }
These are just a few examples of interesting HTML, JavaScript, DOM, and CSS hacks that can help you create more dynamic and sophisticated web experiences. Exploring these techniques can expand your web development toolkit and lead to more creative solutions.
You can create containers that maintain a constant aspect ratio by using padding-bottom as a percentage. The CSS spec says that padding-bottom is defined relative to the *width*, not height, so if you want a 5:1 aspect ratio container you can do something like this:
- <div style="width: 100%; position: relative; padding-bottom: 20%;">
- <div style="position: absolute; left: 0; top: 0; right: 0; bottom: 0;">
- this content will have a constant aspect ratio that varies based on the width.
- </div>
- </div>
This is useful for responsive layouts where you want the width to adjust dynamically but want to keep, say
You can create containers that maintain a constant aspect ratio by using padding-bottom as a percentage. The CSS spec says that padding-bottom is defined relative to the *width*, not height, so if you want a 5:1 aspect ratio container you can do something like this:
- <div style="width: 100%; position: relative; padding-bottom: 20%;">
- <div style="position: absolute; left: 0; top: 0; right: 0; bottom: 0;">
- this content will have a constant aspect ratio that varies based on the width.
- </div>
- </div>
This is useful for responsive layouts where you want the width to adjust dynamically but want to keep, say, square photo thumbnails.
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of th
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of the biggest mistakes and easiest ones to fix.
Overpaying on car insurance
You’ve heard it a million times before, but the average American family still overspends by $417/year on car insurance.
If you’ve been with the same insurer for years, chances are you are one of them.
Pull up Coverage.com, a free site that will compare prices for you, answer the questions on the page, and it will show you how much you could be saving.
That’s it. You’ll likely be saving a bunch of money. Here’s a link to give it a try.
Consistently being in debt
If you’ve got $10K+ in debt (credit cards…medical bills…anything really) you could use a debt relief program and potentially reduce by over 20%.
Here’s how to see if you qualify:
Head over to this Debt Relief comparison website here, then simply answer the questions to see if you qualify.
It’s as simple as that. You’ll likely end up paying less than you owed before and you could be debt free in as little as 2 years.
Missing out on free money to invest
It’s no secret that millionaires love investing, but for the rest of us, it can seem out of reach.
Times have changed. There are a number of investing platforms that will give you a bonus to open an account and get started. All you have to do is open the account and invest at least $25, and you could get up to $1000 in bonus.
Pretty sweet deal right? Here is a link to some of the best options.
Having bad credit
A low credit score can come back to bite you in so many ways in the future.
From that next rental application to getting approved for any type of loan or credit card, if you have a bad history with credit, the good news is you can fix it.
Head over to BankRate.com and answer a few questions to see if you qualify. It only takes a few minutes and could save you from a major upset down the line.
How to get started
Hope this helps! Here are the links to get started:
Have a separate savings account
Stop overpaying for car insurance
Finally get out of debt
Start investing with a free bonus
Fix your credit
Adding label tags around a checkbox or radio button ties that text to the checkbox. No more labelfor!
<label><input type="checkbox" name="option1">I Agree</label>
If the user clicks on "I Agree" it will select that checkbox.
Have you ever see 3D view of DOM in Firefox Developer Tool. It is very cool.
If you inspect an webpage on Firefox using Developer Tool. Click on 3D button will give you a 3D view of all the existing DOM. Use mouse to rotate DOM objects and layers.
Have you ever see 3D view of DOM in Firefox Developer Tool. It is very cool.
If you inspect an webpage on Firefox using Developer Tool. Click on 3D button will give you a 3D view of all the existing DOM. Use mouse to rotate DOM objects and layers.
A lot has been mentioned here. Just to add a few more:
1. in keyword ( All )
- 3 in [1,2,3,4] // => true since index 3 exists (not value)
- Infinity in window // => true for objects
Also interesting to note that arrays are implemented in JS as associative arrays (which is why the example above works) and are more like shortcuts where the keys are indices and the entries are values.
- ["a", "b", "c"]["0"] === { "0": "a", "1": "b", "2": "c" }["0"]
2. Neat array functions ( Chrome, FF )
- [1,2,3,4].reduce(function(x,y) { return x + y; })
- [1,2,3].some(function(x) { return x === 2 });
3. Shorthand anon functions
A lot has been mentioned here. Just to add a few more:
1. in keyword ( All )
- 3 in [1,2,3,4] // => true since index 3 exists (not value)
- Infinity in window // => true for objects
Also interesting to note that arrays are implemented in JS as associative arrays (which is why the example above works) and are more like shortcuts where the keys are indices and the entries are values.
- ["a", "b", "c"]["0"] === { "0": "a", "1": "b", "2": "c" }["0"]
2. Neat array functions ( Chrome, FF )
- [1,2,3,4].reduce(function(x,y) { return x + y; })
- [1,2,3].some(function(x) { return x === 2 });
3. Shorthand anon functions (FF)
- (x) => x*x
4. Conversions (All)
Convert/copy arguments array or NodeList to a real array object:
- function() {
- args = Array.prototype.slice.call(arguments); // real argument array
- }
Unary operator, which converts a string to a number, shorter and faster than parseInt
- +"55" === 55 // => true
Shorter and faster replacement for Math.truncate()
- ~~27.4 === 27
1. You can chain CSS selectors, e.g.:
- <style>
- .myClass { color: green;}
- .myClass.myClass { color: red; }
- </style>
- <div class="myClass">what color am I?</div>
in case you need to be stronger than a selector that is already on the page that you don't have the possibility to change.
2. The Chrome DevTools allow you neat things like:
Access the currently selected element via:console.log($0);
See fired events with:monitorEvents(window,'click');
and kick off the debugger the next time the given function is called:debug(myFunction);
Almost all answers are about Javascript hacks, so i'm putting together a small list of some CSS hacks that i know and use:
The calc() function:
- /* property: calc(expression) */
- .test{
- width: calc(100% - 80px);
- }
- .test2{
- width: calc(100% / 6);
- }
There are really a lot of uses for this function and it will make your life a lot easier. Its supported by most modern browsers to my knowledge, but it doesn't hurt to put a fallback for it just in case.
Child Selectors:
Selectors are widely used these days, but most people don't know the extent of their power:
- /* Basic uses */
- .test:first-child{
- margin-top:20px;
- }
Almost all answers are about Javascript hacks, so i'm putting together a small list of some CSS hacks that i know and use:
The calc() function:
- /* property: calc(expression) */
- .test{
- width: calc(100% - 80px);
- }
- .test2{
- width: calc(100% / 6);
- }
There are really a lot of uses for this function and it will make your life a lot easier. Its supported by most modern browsers to my knowledge, but it doesn't hurt to put a fallback for it just in case.
Child Selectors:
Selectors are widely used these days, but most people don't know the extent of their power:
- /* Basic uses */
- .test:first-child{
- margin-top:20px;
- }
- .test:last-child{
- margin-top:30px;
- }
- /* More advanced uses */
- .test:nth-child(2){
- margin-top:5px;
- }
- .test:nth-child(5){
- margin-top:10px;
- }
- /* Very advanced uses */
- .test:not(p){ /* Style all the elements that are not a <p> */
- display:none;
- }
- p:last-of-type{ /* Style the last element of type <p> */
- border-bottom:1px solid #ff0;
- }
- tr:nth-child(even){ /* Style all the elements that have an even index */
- background: #CCC
- }
- tr:nth-child(odd){ /* Style all the elements that have an odd index */
- background: #fff
- }
- /* Select a range of children */
- tr:nth-child(n+3):nth-child(-n+5){
- background:#fff /* will selects only children 3, 4 and 5. */
- }
Selectors that most people don't know about or use:
- :disabled Selects every disabled <input> element.
- :empty Selects every <p> element that has no children (including text nodes).
- :first-letter Selects the first letter of every element.
- :first-line Selects the first line of every element.
- :lang(fr) Selects every element with a lang attribute equal to "fr" (French).
There are a lot of other selectors but i assume that most well versed developers already know about them.
Cross-browser transparency:
Adding a transparent div sometimes is needed in a website but its a pain because most the known property are not cross-browser, by implementing this code it become cross-browser.
- .transparent{
- filter:alpha(opacity=50);
- -moz-opacity:0.5;
- -khtml-opacity: 0.5;
- opacity: 0.5;
- }
Set color of the selected text:
I don't know about the browser compatibility for this one, but its a pretty cool trick:
- ::selection{
- background: #ffb7b7;
- }
Conditional Style-sheets:
- <!--[if lte IE 8]><link rel="stylesheet" href="lte-ie-8.css"><![endif]-->
- <!--[if lte IE 7]><link rel="stylesheet" href="lte-ie-7.css"><![endif]-->
- <!--[if lte IE 6]><link rel="stylesheet" href="lte-ie-6.css"><![endif]-->
This is a very useful trick, its somewhat known and for HTML not CSS, but i decided to add it, just in case.
This is all i can think of right now, bit i'm sure i forgot to put a couple of tricks and hacks. Once i remember something new, i'll comeback and edit my answer. Feel free to leave a comment if you have any questions.
You can make a triangle with just CSS(just like the tiny triangle on Vimeo sidebar).
- .triangle {
- width: 0;
- height: 0;
- border-top: 100px solid #0099ff;
- border-left: 100px dashed transparent;
- border-right: 100px dashed transparent;
- border-bottom: 0;
- display: block;
- overflow: hidden;
- }
Demo: http://leplay.github.com/demo/triangle.html
Update: fix bugs in fucking IE6, and add a new demo.
Hello, i will Provide the Rarest ones. but Firstly you need to know what some terms means.
First of All:
Browser Compatibility Checking:
Can I use... Support tables for HTML5, CSS3, etc
*Global usage share statistics based on data from StatCounter GlobalStats for August, 2015. See the browser usage table for usage by browser version.
Polyfill:
In web development, a polyfill (or polyfiller) is downloadable code which provides facilities that are not built into a web browser. It implements technology that a developer expects the browser to provide natively, providing a more uniform API landscape.
F
Hello, i will Provide the Rarest ones. but Firstly you need to know what some terms means.
First of All:
Browser Compatibility Checking:
Can I use... Support tables for HTML5, CSS3, etc
*Global usage share statistics based on data from StatCounter GlobalStats for August, 2015. See the browser usage table for usage by browser version.
Polyfill:
In web development, a polyfill (or polyfiller) is downloadable code which provides facilities that are not built into a web browser. It implements technology that a developer expects the browser to provide natively, providing a more uniform API landscape.
Fallback:
Code fallbacks are the perfect solution for compromising with your many unique visitors. Not everybody on the web is using the same operating system, web browser, or even physical hardware. All of these factor into how your web page will actually render on screen. When working with new CSS or JavaScript tricks you’ll often run into such technical bugs and compatibility issues, so you need to use some fallback techniques to fix them.
[CSS] @supports - use it, be cautious
Feature detection has always been a good practice, but unfortunately we could only do that kind of thing using JavaScript. But not anymore.
CSS Feature Queries allow authors to condition rules based on whether particular property declarations are supported in CSS using the @supports at rule.
For now it's available in FF 22+, Opera 12.1+, and Chrome 28+.
[API] Vibration - use it, be cautious
Vibration enables web apps access to a supporting device's force feedback motor.
The Vibration API is specifically targeted towards the gaming use case, and not intended for activities such as notifications or alerts on a user's mobile phone for example.
Being a mobile-based feature, the unprefixed feature is supported in Firefox for Android,Firefox OS, Chromium 32, and so in Chrome 32+ on Android and Opera 20+ on Android. Safari Mobile and IE mobile do not support it yet.
[HTML] WebP - use it, be cautious
WebP is an image format that's designed to be provide high quality photographic imagery through smaller filesizes than JPEG. It's supported natively in Chrome, Android, and Opera.
WebP alpha support is available in Chrome 22 (along with lossless). You can detect alpha support with a onload/onerror handlers.
For now, Modernizr detects WebP support and you can manage your own fallback to jpeg.
Recommended polyfills: WebPJS
[API] Web Workers - you can use it, with fallback
Web Workers can parallelize computationally heavy operations, freeing up the UI thread to respond to user input.
For browsers that do not support web workers, depending on your use case, you may choose not to offer the feature that web workers provide or to chunk it up (http://www.nczonline.net/blog/2009/01/13/speed-up-your-javascript-part-1/) and put it on the UI thread.
[API] WebGL - use it, be cautious
There are polyfills and plugins to enable WebGL for IE, but you probably shouldn't use them. Use WebGL if it's present, else point the user to get.webgl.org.
WebGL is used to make game like Minecraft and android games.. in the Browser (OpenGL for the Web)
[HTML] <track> - you can use it, with polyfill
The <track> element provides a mechanism of showing captions/subtitles and/or associated metadata with <audio> or <video>. The file format the <track> element uses is called WebVTT. Browser support for both is in progress: complete in IE10pp4, ongoing in Webkit, unknown in Opera. A polyfill is a wise choice; Captionator is the most complete and well supported. More at Sylvia Pfeiffer's blog.
Recommended polyfills:
captionator.js
[HTML] <ruby> - you can use it, with fallback
If you are using the Ruby element you don't need a script-based fallback, but you can provide a CSS-based set of good defaults.
Recommended polyfills: Cross-browser Ruby
[API] requestAnimationFrame - you can use it, with polyfill
requestAnimationFrame is recommended for animation as it's battery and power friendly and allows the browser to optimize the performance of your animations.
The spec has gotten some fixes and settled down. All major browsers currently supportrequestAnimationFrame. If you need full browser support, be sure to use the lightweight polyfill.
An interesting usecase: If you have vertical parallax that changes on scroll, you can consider using rAF instead of binding to a window's scroll event. In this way, you'd just ask forwindow.scrollTop on your rAF callback and take action. This will give you the smoothest animations possible.
Recommended polyfills: requestAnimationFrame polyfill
[CSS] regions - avoid
CSS Regions is in active development. As a result, the syntax is in flux. A polyfill based on an older syntax exists, but we recommend you hold your horses till this spec sees some stability and 3 or more implementations.
[CSS] position: sticky - you can use it with polyfill, be cautious
position: sticky combines aspects of relative and fixed positioning. Elements to which it is applied initially act as though they are position: relative, but switch to acting likeposition: fixed when they reach a specified position relative to the viewport. This behavior is often useful for section headings or navigational sidebars.
This Mozilla presentation video
and
this live demo of the Fixed-sticky polyfill
show how position: sticky behaves in common use-cases.
Supported unprefixed in Firefox 30.0+. Supported with a prefix (as -webkit-sticky) in OS X Safari 7.0+ and iOS Safari 6.0+. Currently part of the
CSS Positioned Layout Module Level 3 W3C Working Draft
.
Caution is advised since the spec is currently only at the Working Draft stage and thus can be subject to change. The usage of a polyfill is strongly recommended due to the current level of browser support. Signs currently seem positive for browser support increasing in the future.
Use
Fixed-sticky
for simple top or bottom aligned stickies. Use
Stickyfill
for a wider range of use cases with top aligned sticky positioned blocks and table cells.
Recommended polyfills: Fixed-sticky, Stickyfill
- There much more.. but this ones are rare. Please feel free to ask and follow me :)
If you have any comment, suggestion, recommendation or correction please feel free to comment.
You can prevent your website from being loaded as an iframe in someone else's site with this JS statement:
- if ( window.location != window.parent.location )
- window.parent.location = window.location;
Using the attribute selector is tantamount to sorcery:
Select all <a> that are hrefs:a[href]{}
Select all elements that have a class (useful if JS is adding classes somewhere)article[class]{}
Select an ID, but with the specificity of a class:
- section[id="sideBar"]{color: red}
Style all links that are fully qualified URLs, knowing that ^ means "starts with":
- a[href^="http"]{}
Need to style a link to a ZIP or a PDF? Totally fine, too. Use the $ which means "ends with"
a[href$=".zip"]:after{content:"(zip)"}
Style an image based on one word existing in the alt attribute? Let's use the space substrin
Using the attribute selector is tantamount to sorcery:
Select all <a> that are hrefs:a[href]{}
Select all elements that have a class (useful if JS is adding classes somewhere)article[class]{}
Select an ID, but with the specificity of a class:
- section[id="sideBar"]{color: red}
Style all links that are fully qualified URLs, knowing that ^ means "starts with":
- a[href^="http"]{}
Need to style a link to a ZIP or a PDF? Totally fine, too. Use the $ which means "ends with"
a[href$=".zip"]:after{content:"(zip)"}
Style an image based on one word existing in the alt attribute? Let's use the space substring selector:
- img[alt~="Insurance"]{}
Style an image based on the name of the image file itself? Also doable, even if you're image is this-image-shot-april-2-2014.png. The | is a hyphen substring selector:
- img[src|="-april-"]{}
There's some even more crazy stuff that you can do with the attribute selector, which I wrote about here: CSS Sorcery: Performing Magic with the Attribute Selector
There is a CSS property for calculating width and height called calc. Here is an example:
Element {
Width:calc(100% - 50px);
}
This sets the elements width to be 100% of the parent - 50px. It is helpful for making sites mobile friendly. It also has good browser support (http://caniuse.com/#feat=calc).
Another technique EDIT:
To make an element the exact height and width of the browser window, I like to use this but of JavaScript:
document.getElementById('element').style.height = window.innerHeight+'px';
document.getElementById('element').style.width = window.innerWidth+'px';
If you execute that cod
There is a CSS property for calculating width and height called calc. Here is an example:
Element {
Width:calc(100% - 50px);
}
This sets the elements width to be 100% of the parent - 50px. It is helpful for making sites mobile friendly. It also has good browser support (http://caniuse.com/#feat=calc).
Another technique EDIT:
To make an element the exact height and width of the browser window, I like to use this but of JavaScript:
document.getElementById('element').style.height = window.innerHeight+'px';
document.getElementById('element').style.width = window.innerWidth+'px';
If you execute that code onload and onresize then it will always be the exact width and height of the browser window.
Of course for setting the width, this CSS usually works:
Element {
width:100%;
}
Not exactly a hack...
On Chrome you can pretty-print an associative array to the console using console.table(arr)
I don't know if this works in other browsers.
Example:
- var teams_data = [{team: 'Chelsea', goals: 4}, {team: 'Man. Utd', goals: 3}];
- console.table(teams_data);
Vertically center a content block:
(IE9+)
- .center-vertical {
- position: relative;
- top: 50%;
- transform: translateY(-50%);
- }
Horizontally center a content block:
(IE9+)
When centering content that is wider than its parent, like a landscape image for example, margin: 0 auto wi...
There are two units in CSS that have a solid browser support, yet they are widely underused:
vh & vw (viewport width & viewport height)
One of the scenarios where we can use vh is a website with about 3-4 scrollable pages of content on which we have some elements. If we want to make each element the same height as the viewport (not the body height), the height: 100%; will not do the trick. Instead, height: 100vh; on each element will give each one the height of the viewport size.
To prevent the content from being hiddden if it overflows the height of that element, a min-height can be used.
There are two units in CSS that have a solid browser support, yet they are widely underused:
vh & vw (viewport width & viewport height)
One of the scenarios where we can use vh is a website with about 3-4 scrollable pages of content on which we have some elements. If we want to make each element the same height as the viewport (not the body height), the height: 100%; will not do the trick. Instead, height: 100vh; on each element will give each one the height of the viewport size.
To prevent the content from being hiddden if it overflows the height of that element, a min-height can be used.
To make URLs automatically load from either 'http://' or 'https://' based on the current protocol, you can write them like this:
- <script src="//domain.com/path/to/script.js"></script>
By setting the font-size of the <body> (which by default its 16px) to 62.5%, setting em based font-sizes for the rest of the page becomes very simple. just divide the intended pixel size by 10 to get the equivalent size in ems.
10px = 1.0 em
11px = 1.1em
12px = 1.2em
etc ....
This becomes extremely useful when converting an entire site from px to ems
note: This also works exactly the same with rem units as well (they are interchangeable with this trick). As Quora User pointed out, its probably better to use rems.
This statement will print a complete Javascript stack trace in the console of any modern browser, including all named and anonymous functions complete with arguments and values. Use it within any method to see the call stack to that point:
- console.trace();
CSS has vw and vh units that correspond to the browser viewport rather than the document size: vw refers to the window width and vh is the height and they are always 1/100 of each dimension. You can make something be the exact height of the viewport/window easily with this:
- .makeItWindowHeight {
- height: 100vh;
- }
More info:
This statement will print a complete Javascript stack trace in the console of any modern browser, including all named and anonymous functions complete with arguments and values. Use it within any method to see the call stack to that point:
- console.trace();
CSS has vw and vh units that correspond to the browser viewport rather than the document size: vw refers to the window width and vh is the height and they are always 1/100 of each dimension. You can make something be the exact height of the viewport/window easily with this:
- .makeItWindowHeight {
- height: 100vh;
- }
More info:
Some people doesn't know, but everything in JS is object.
Some people think that numbers isn't objects because you can't do something like 1.toString().
But they doesn't know that full syntax for numbers is 1. (with dot) and 1 (without dot) is short syntax. You can write something like 0.5 == .5 == 1. / 2 and also you can write 1..toString() and it will work! lol
HTML is an attractive language .a beginner or a non coder can easily learn this language easily. I suggest W3 schools to learn about these languages.
Here are some of the hacks about HTML, CSS, JS:-
1. Use file system to cache resources:-
• Copy file from my Windows to Sandbox.
• Create offline mode for content eg:-email ,video.
2. Use either visual studio code or notepad for HTML codes.
3. Use <picture> element to improve image styling.
4. Take advantage for SEO.
5. Local storage is better instead of cookies.
6. Use the File System API to CACHE media Resources locally.
Html5 filesystem API easy conceiv
HTML is an attractive language .a beginner or a non coder can easily learn this language easily. I suggest W3 schools to learn about these languages.
Here are some of the hacks about HTML, CSS, JS:-
1. Use file system to cache resources:-
• Copy file from my Windows to Sandbox.
• Create offline mode for content eg:-email ,video.
2. Use either visual studio code or notepad for HTML codes.
3. Use <picture> element to improve image styling.
4. Take advantage for SEO.
5. Local storage is better instead of cookies.
6. Use the File System API to CACHE media Resources locally.
Html5 filesystem API easy conceived as an alternative to app cache to enable dynamic caching of assets. but did you know that you can also use it to support interactions with files stored on the user’s local device.
window.setTimeout(function(){},0) actually takes ~10-20 ms, if you want to yield but run immediately after, you can use window.postMessage and add a listener to run the function. (Note: I've been corrected in the comments that its more like 4 ms now, but this method is still faster when you really need the speed boost).
To parse a JSON blob, you can run eval(), but for some browsers it's much, much faster to do this:
[code=js]var jsonobject = new Function('return ' + jsonstring)();[/code]
You can make the browser escape html for you:
[code=js]var div = document.createElement('div');
div.innerTe
window.setTimeout(function(){},0) actually takes ~10-20 ms, if you want to yield but run immediately after, you can use window.postMessage and add a listener to run the function. (Note: I've been corrected in the comments that its more like 4 ms now, but this method is still faster when you really need the speed boost).
To parse a JSON blob, you can run eval(), but for some browsers it's much, much faster to do this:
[code=js]var jsonobject = new Function('return ' + jsonstring)();[/code]
You can make the browser escape html for you:
[code=js]var div = document.createElement('div');
div.innerText = '<script>alert("hello world");<\/script>';
div.innerHTML
// returns '<script>alert("hi");</script>'[/code]
You can also make the browser unescape html for you, but you need to do a bit more hacking:
[code=js]function unescape(html) {
var div = document.createElement('div');
div.innerHTML = html.replace(/</g, '<');
return div.innerText;
}[/code]
I also wrote something about different communication options in JavaScript: http://www.benmcmahan.com/javascript/transport.html
CSS3 Blur text:
- color: transparent;
- text-shadow: #111 0 0 5px;
http://jsbin.com/welcome/23340/
You can easily check all global variables by just console logging:
keys(window);
I keep a file called SurprisesAboutJavaScript.txt because I sometimes find odd little corners I want to remember. Here's some of it:
Explicitly naming an anonymous function gives you a variable, scoped to that function, that allows it to refer to itself--sort of like 'this' but for the function itself. This is called an "inline named function", per the book Secrets of the JavaScript Ninja, section 4.2.4.
- // the external handle is 'x.myMethod', but the function
- // recursively calls itself via its inline name, 'signal'
- var x = {
- myMethod: function signal(n) {
- return n > 1 ? signal(n-1) +
I keep a file called SurprisesAboutJavaScript.txt because I sometimes find odd little corners I want to remember. Here's some of it:
Explicitly naming an anonymous function gives you a variable, scoped to that function, that allows it to refer to itself--sort of like 'this' but for the function itself. This is called an "inline named function", per the book Secrets of the JavaScript Ninja, section 4.2.4.
- // the external handle is 'x.myMethod', but the function
- // recursively calls itself via its inline name, 'signal'
- var x = {
- myMethod: function signal(n) {
- return n > 1 ? signal(n-1) + "-beep" : "beep";
- }
- };
Each function has a property, 'length', whose sole purpose is to hold the number of parameters the function was originally defined with. This lets you compare the length of 'arguments' to the length of the current function to find out whether it is being called with the original number of parameters. (from Secrets of the JavaScript Ninja)
When running a compatibility check, you can save cycles after the first run of a function if that function overwrites itself on first execution. (from High Performance JavaScript)
- function addHandler(target, eventType, handler) {
- // overwrite the existing function
- if (target.addEventListener) { // DOM2 Events
- addHandler = function(target, eventType, handler) {
- target.addEventListener(eventType, handler, false);
- };
- }
- else { // assume IE
- addHandler = function(target, eventType, handler) {
- target.attachEvent("on" + eventType, handler);
- };
- }
- // call the new function
- addHandler(target, eventType, handler);
- }
Double-negating something with !!
will convert it to the equivalent boolean primitive (due to the way JS coerces each side of a comparison):
if (!!1 === true) { console.log('Yep.'); }
The property document.defaultView
is a shortcut to the Head/Global object. That's 'window' in a browser, but if you're dealing with a headless browser or a js environment outside a browser, you can use this to get access to the head object scope. From the book DOM Enlightenment.
There's more, but I don't want to be all spammy with my notes file. :) I particularly like the combination of "you can store arbitrary text content inside a script tag" and "you can eval
arbitrary text content".
UPDATE: Since a few folks have asked, here's a bit more--this is detail on how you can store and eval code chunks as text. There's lots of uses for the technique, but at the most basic level it lets you load required javascript as a page resource without actually running it through the interpreter. That lets you execute if it becomes necessary, but doesn't require an additional network call (since it's already in the browser's memory as part of the DOM).
So: you can store arbitrary js in a script tag with a non-standard type attribute, and the browser will ignore it. You can then pull it in and evaluate it in other code. (both parts of this are from JS Ninja, Chapter 9):
- <script type="text/javascript">
- window.onload = function() {
- var scripts = document.getElementsByTagName('script');
- for (var i = 0; i < scripts.length; i++) {
- if (scripts[i].type = 'x/onload') {
- globalEval(scripts[i].innerHTML);
- }
- }
- };
- </script>
- <script type="x/onload">
- assert(true, "Executed on page load.");
- </script>
Then you can force evaluation of runtime strings in the global scope in a browser safe manner like this:
- function globalEval(data) {
- data = data.replace(/^\s*|\s*$/g, "");
- if (data) {
- var head = document.getElementsByTagName("head")[0] ||
- document.documentElement,
- script = document.createElement('script');
- script.type = 'text/javascript';
- script.text = data;
- head.appendChild(script); // attach to the DOM
- head.removeChild(script); // remove from the DOM
- }
- }
Use download attribute with file download links, like: <a href="fileurl" download="report.pdf">download></a>
when you need to place download link on your site. Without download
attribute backend developer have to add some special HTTP-headers to response in order to start downloading process. Also, you can specify user-friendly filename (like 'report.doc', 'cv.pdf', etc), when you store files with long, unreadable names.
I recently discovered that I can set the URL bar in the browser to something else. This is useful if you are calling an internal URL in your application, but want that your users use another URL for public sharing.
- function setURLBar(theurl) {
- history.pushState({}, '', theurl);
- }
Blew my mind ;-)
There's a CSS property that allows you to load in attribute contents from the HTML element and it's supported in most browsers, even by IE8.
Add percentage value after a range slider input field:<input id="slider" type="range" min="0" max="100" value="50">
- #slider:after {
- content: attr(value) "%";
- // custom styling
- }
Add numbering after a notification button (Facebook style) with CSS only:<button id="inbox" type="button" data-new-items="8">Inbox</button>
- #inbox:after {
- content: attr(data-new-items);
- // custom styling
- }
Not really a hack, but many people I worked with didn't know that you can pass more than one argument to console.log()
separated by comma:
console.log(foo, bar, baz)
One big advantage over writing console.log(foo + bar + baz)
is that passed arguments aren't type converted.
- var a = 1, b = "2", c = 3;
- console.log(a, b, c);
- console.log(a + b + c);
results in:
- 1 "2" 3
- 123
Another one: in Chrome Devtools' console (and I think in Firefox too) $0
is a shortcut to the currently selected element in the elements panel.
// edit:
Not exactly useful for development but pretty handy though: in chrome you can find o
Not really a hack, but many people I worked with didn't know that you can pass more than one argument to console.log()
separated by comma:
console.log(foo, bar, baz)
One big advantage over writing console.log(foo + bar + baz)
is that passed arguments aren't type converted.
- var a = 1, b = "2", c = 3;
- console.log(a, b, c);
- console.log(a + b + c);
results in:
- 1 "2" 3
- 123
Another one: in Chrome Devtools' console (and I think in Firefox too) $0
is a shortcut to the currently selected element in the elements panel.
// edit:
Not exactly useful for development but pretty handy though: in chrome you can find out a saved password pretty easily without looking for it in the settings by just right-clicking the password field (with the password filled in), then "inspect element" and then in the dev console just enter: $0.value
(look above to find out what $0
stands for ;))
You can assign a core JS function into another variable to preserve a reference to it, overwrite the core function with your own and call the original one inside your custom function.
For example, tracing the number of times someone left an alert in their code:
- (function () {
- var oldAlert = window.alert,
- count = 0;
- window.alert = function (a) {
- count++;
- oldAlert(a+"\n You've called alert "+count+" times now. Stop, it's evil!");
- };
- })();
- alert("Hello World");
Have fun!
1. You can pass arguments into setTimeout and setInterval
Say you want to pass in two variables to the function you call in setTimeout. You'd probably think this is the only way to do it:
var callback = function(a,b){
console.log(a + b); // 'foobar'
}
window.setTimeout(function(){
callback('foo', 'bar');
}, 1000);
But you can accomplish the same thing with this:
window.setTimeout(callback, 1000, 'foo', 'bar');
Note that this isn't supported on IE (not even IE9).
2. Old String functions
If you type in String.prototype into your console, you can see a bunch of old functions you can use to wrap the text in H
1. You can pass arguments into setTimeout and setInterval
Say you want to pass in two variables to the function you call in setTimeout. You'd probably think this is the only way to do it:
var callback = function(a,b){
console.log(a + b); // 'foobar'
}
window.setTimeout(function(){
callback('foo', 'bar');
}, 1000);
But you can accomplish the same thing with this:
window.setTimeout(callback, 1000, 'foo', 'bar');
Note that this isn't supported on IE (not even IE9).
2. Old String functions
If you type in String.prototype into your console, you can see a bunch of old functions you can use to wrap the text in HTML tags (some deprecated HTML obviously):'foo'.italics()
...will return this:'<i>foo</i>'
Similarly for strike(), sub(), small(), link() (Brian Yee refers to this in his comment), etc.
3. An HTML element with an ID creates a JavaScript global with the same name
Surprising but true, and it's done by modern browsers as a means of backwards compatibility:HTML:
<div id="someInnocentDiv"></div>
JS:
console.log(someInnocentDiv); // <div id="someInnocentDiv"></div>
4. You can read the text at any XY coordinate on a webpage
var x = 50, y = 100;
var range = document.caretRangeFromPoint(x, y);
if(range) {
range.expand('word');
var word = range.startContainer.textContent.substring(range.startOffset, range.endOffset);
console.log(word);
}
A cross-browser compatible way of doing this: Edit fiddle - JSFiddle - Code Playground. You can base64 encode files dropped onto the document from your desktop
document.ondrop = function (e) {
e.preventDefault(); // prevent browser from trying to run/display the file
var reader = new FileReader();
reader.onload = function(e) {
console.log(e.target.result); // base64 encoded file data!
};
reader.readAsDataURL(e.dataTransfer.files[0]);
}
True Element Blur (without images/shadows)
- .blur {
- -webkit-filter: blur(4px);
- -moz-filter: blur(4px);
- filter: blur(4px);
- }
Currently only supported in Webkit based browsers. (CSS Filter Effects)
I think <datalist>
tag is interesting and not well known. This tag specifies a list of predefined options for an <input>
element. The <datalist>
tag is used to provide an "autocomplete" feature on <input>
elements. Users will see a drop-down list of pre-defined options as they input data.
<input list="browsers">
- <datalist id="browsers">
- <option value="Internet Explorer">
- <option value="Firefox">
- <option value="Chrome">
- <option value="Opera">
- <option value="Safari">
- </datalist>
Use the <input>
element's list attribute to bind it together with a <datalist>
element
src: HTML datalist Tag
While the term "hack" can sometimes imply inelegant solutions, there are definitely some lesser-known techniques in HTML/JS/DOM/CSS that can be surprisingly powerful and creative. Here are a few interesting options to explore:
CSS Houdini Magic:
- Custom Properties (CSS Variables): These aren't exactly unknown, but their power goes beyond simple theming. You can use them for calculations, animations, and even responsive design tricks. Imagine a variable holding your font size, and then using it to dynamically adjust margins based on screen size.
JavaScript for Unexpected Effects:
- Using
requestAnimat
While the term "hack" can sometimes imply inelegant solutions, there are definitely some lesser-known techniques in HTML/JS/DOM/CSS that can be surprisingly powerful and creative. Here are a few interesting options to explore:
CSS Houdini Magic:
- Custom Properties (CSS Variables): These aren't exactly unknown, but their power goes beyond simple theming. You can use them for calculations, animations, and even responsive design tricks. Imagine a variable holding your font size, and then using it to dynamically adjust margins based on screen size.
JavaScript for Unexpected Effects:
- Using
requestAnimationFrame
for smooth animations: This leverages the browser's refresh cycle for buttery-smooth animations, a clear step up from traditionalsetInterval
.
DOM Manipulation Wizardry:
- Shadow DOM: This isolates DOM subtrees, allowing for encapsulated styling and functionality. It can be a powerful tool for building reusable components.
HTML Semantics for Hidden Gems:
- The
<details>
and<summary>
elements: These create interactive collapsible sections, perfect for accordions or hiding/revealing additional content.
Creative Combinations:
- **Using CSS
content
property with pseudo-elements (:before
and:after
) to create shapes and design elements purely with CSS. This can be surprisingly effective for lightweight decorations or UI elements.
Remember:
- These techniques can be complex and have browser compatibility considerations. It's good practice to test thoroughly and use them judiciously.
- While some "hacks" might solve a specific problem, focus on well-established standards whenever possible for maintainable and accessible code.
Finding More Hacks:
- Explore CodePen collections or follow developers known for creative CSS solutions. There's a whole community out there pushing the boundaries!
Remember, the best hacks are often clever solutions to specific problems. Keep your eyes peeled for these techniques and experiment responsibly to expand your web development toolkit!
You can change the browser's URL without reloading using Pushstate.
This is very useful in making the browser history work for ajax powered apps, as well as making its links shareable. See it in action when your browsing the source on Github.com.
JavaScript
The bind method is very useful for creating shortcut method names that maintain their "this" reference.
So in console.log, "this" in the log method is console.
var log = console.log.bind(console);
Now you have a shorter console.log without a function wrapper.
Paste this code into your browser's address bar and press enter.
- javascript: document.body.contentEditable ='true'; document.designMode='on'; void 0
Now, you can edit the content of your webpage!
Catch an exception without a 'catch' statement:
- function test () {
- try {
- throw new Error ('oops');
- }
- finally {
- // drop the exception!
- return;
- }
- }
- // no exception will be thrown
- test ();
This happens because the 'finally' statement can 'override' the control flow. An interesting detail many developers never know.